本文由 发布,转载请注明出处,如有问题请联系我们! 发布时间: 2021-08-01hbase常用命令及使用方法-查看hbase表结构的命令
加载中最先根据docker安装Hbase。
docker search hbase docker pull harisekhon/hbase开启hbase镜像系统。
docker run -d -p 2181:2181 -p 8080:8080 -p 8085:8085 -p 9090:9090 -p 9095:9095 -p 16000:16000 -p 16010:16010 -p 16201:16201 -p 16301:16301 -p 16030:16030 -p 16020:16020 --name hbase001 harisekhon/hbase浏览hbase。
浏览localhost:16010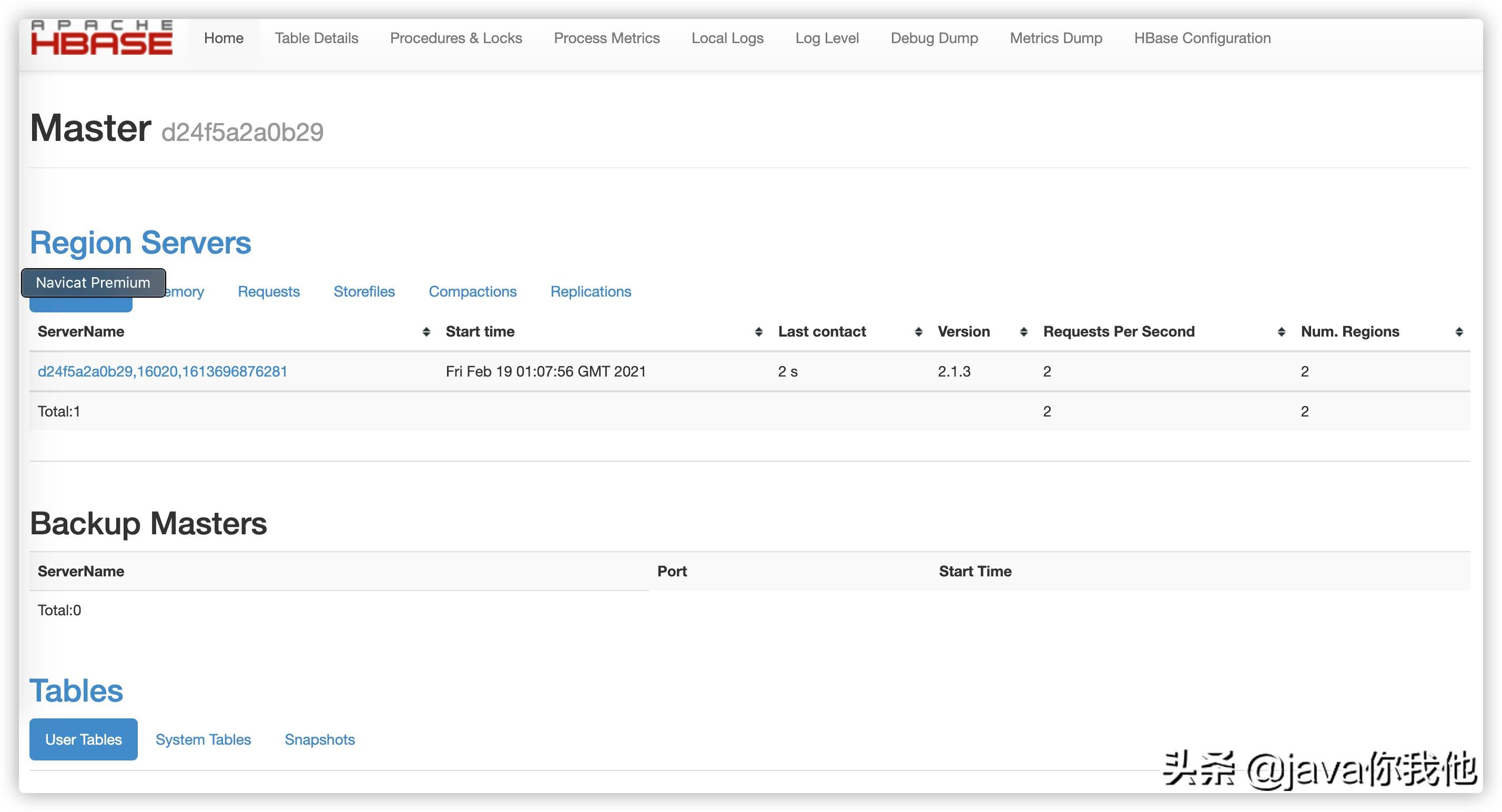
进行以上指令实际操作后,大家进入了hbase的cmd实际操作方式:
下面,大家建立一个名字室内空间为default的表,表名叫test,列族为cf: create' test ',' cf '全部表都能够根据list指令:list查询。
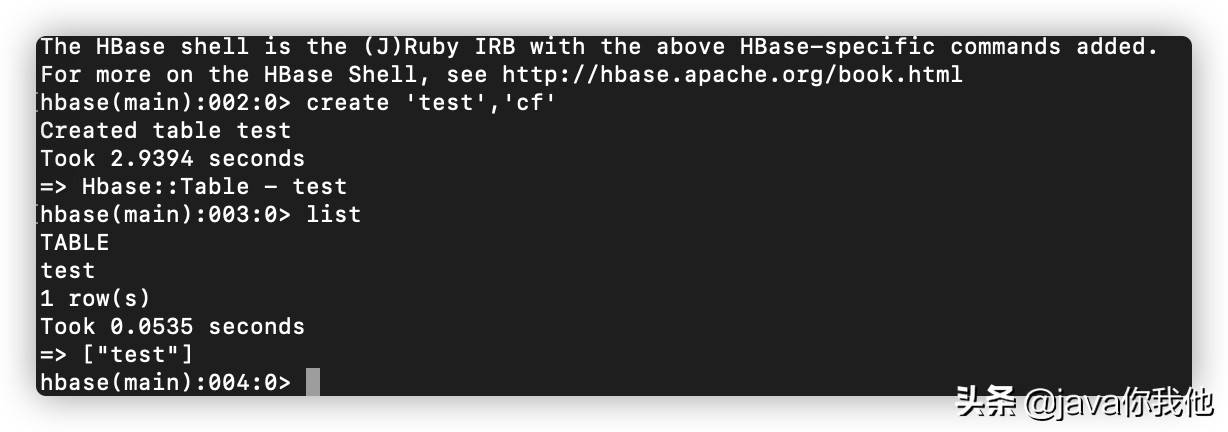
下边是怎样根据java api实际操作hbase。
加上maven依靠 org.apache.hbase hbase-client 2.1.3检测工具栏如下所示:
package com.example.redisiondemo.utils;import org.apache.hadoop.conf.Configuration;import org.apache.hadoop.hbase.*;import org.apache.hadoop.hbase.client.*;import org.apache.hadoop.hbase.client.Admin;import org.apache.hadoop.hbase.util.Bytes;import java.io.IOException;import java.util.ArrayList;import java.util.List;public class HbaseTest { private static Admin admin; private static final String COLUMNS_FAMILY_1 = "cf1"; private static final String COLUMNS_FAMILY_2 = "cf2"; public static Connection initHbase() throws IOException { Configuration configuration = HBaseConfiguration.create(); configuration.set("hbase.zookeeper.quorum", "127.0.0.1"); configuration.set("hbase.zookeeper.property.clientPort", "2181"); configuration.set("hbase.master", "127.0.0.1:16010"); Connection connection = ConnectionFactory.createConnection(configuration); return connection; }//创建表 create public static void createTable(TableName tableName, String[] cols) throws IOException { admin = initHbase().getAdmin(); if (admin.tableExists(tableName)) { System.out.println("Table Already Exists!"); } else { HTableDescriptor hTableDescriptor = new HTableDescriptor(tableName); for (String col : cols) { HColumnDescriptor hColumnDescriptor = new HColumnDescriptor(col); hTableDescriptor.addFamily(hColumnDescriptor); } admin.createTable(hTableDescriptor); System.out.println("Table Create Successful"); } } public static TableName getTbName(String tableName) { return TableName.valueOf(tableName); } // 删除表 drop public static void deleteTable(TableName tableName) throws IOException { admin = initHbase().getAdmin(); if (admin.tableExists(tableName)) { admin.disableTable(tableName); admin.deleteTable(tableName); System.out.println("Table Delete Successful"); } else { System.out.println("Table does not exist!"); } } //put 插进数据信息 public static void insertData(TableName tableName, Student student) throws IOException { Put put = new Put(Bytes.toBytes(student.getId())); put.addColumn(Bytes.toBytes(COLUMNS_FAMILY_1), Bytes.toBytes("name"), Bytes.toBytes(student.getName())); put.addColumn(Bytes.toBytes(COLUMNS_FAMILY_1), Bytes.toBytes("age"), Bytes.toBytes(student.getAge())); initHbase().getTable(tableName).put(put); System.out.println("Data insert success:" student.toString()); } // delete 删掉数据信息 public static void deleteData(TableName tableName, String rowKey) throws IOException { Delete delete = new Delete(Bytes.toBytes(rowKey)); // 特定rowKey// delete = delete.addColumn(Bytes.toBytes(COLUMNS_FAMILY_1), Bytes.toBytes("name")); // 特定column,还可以不特定,删掉该rowKey的全部column initHbase().getTable(tableName).delete(delete); System.out.println("Delete Success"); } // scan数据信息 public static List allScan(TableName tableName) throws IOException { ResultScanner results = initHbase().getTable(tableName).getScanner(new Scan().addFamily(Bytes.toBytes("cf1"))); List list = new ArrayList(); for (Result result : results) { Student student = new Student(); for (Cell cell : result.rawCells()) { String colName = Bytes.toString(cell.getQualifierArray(), cell.getQualifierOffset(), cell.getQualifierLength()); String value = Bytes.toString(cell.getValueArray(), cell.getValueOffset(), cell.getValueLength()); } } return null; } // 依据rowkey get数据信息 public static Student singleGet(TableName tableName, String rowKey) throws IOException { Student student = new Student(); student.setId(rowKey); Get get = new Get(Bytes.toBytes(rowKey)); if (!get.isCheckExistenceOnly()) { Result result = initHbase().getTable(tableName).get(get); for (Cell cell : result.rawCells()) { String colName = Bytes.toString(cell.getQualifierArray(), cell.getQualifierOffset(), cell.getQualifierLength()); String value = Bytes.toString(cell.getValueArray(), cell.getValueOffset(), cell.getValueLength()); switch (colName) { case "name": student.setName(value); break; case "age": student.setAge(value); break; default: System.out.println("unknown columns"); } } } System.out.println(student.toString()); return student; } // 查看特定Cell数据信息 public static String getCell(TableName tableName, String rowKey, String cf, String column) throws IOException { Get get = new Get(Bytes.toBytes(rowKey)); String rst = null; if (!get.isCheckExistenceOnly()) { get = get.addColumn(Bytes.toBytes(cf), Bytes.toBytes(column)); try { Result result = initHbase().getTable(tableName).get(get); byte[] resByte = result.getValue(Bytes.toBytes(cf), Bytes.toBytes(column)); rst = Bytes.toString(resByte); } catch (Exception exception) { System.out.printf("columnFamily or column does not exists"); } } System.out.println("Value is: " rst); return rst; } public static void main(String[] args) throws IOException{ Student student = new Student(); student.setId("1"); student.setName("hzp"); student.setAge("18"); String table = "student"; // createTable(getTbName(table), new String[]{COLUMNS_FAMILY_1, COLUMNS_FAMILY_2}); // deleteTable(getTbName(table)); insertData(getTbName(table), student);// deleteData(getTbName(table), "1"); // singleGet(getTbName(table), "2"); // getCell(getTbName(table), "1", "cf1", "name"); }}student实体线package com.example.redisiondemo.utils;public class Student { private String id; private String name; private String age; public String getId() { return id; } public void setId(String id) { this.id = id; } public String getName() { return name; } @Override public String toString() { return "Student{" "id='" id ''' ", name='" name ''' ", age='" age ''' '}'; } public void setName(String name) { this.name = name; } public String getAge() { return age; } public void setAge(String age) { this.age = age; }}